Jest For Everyone: Write Your First Test
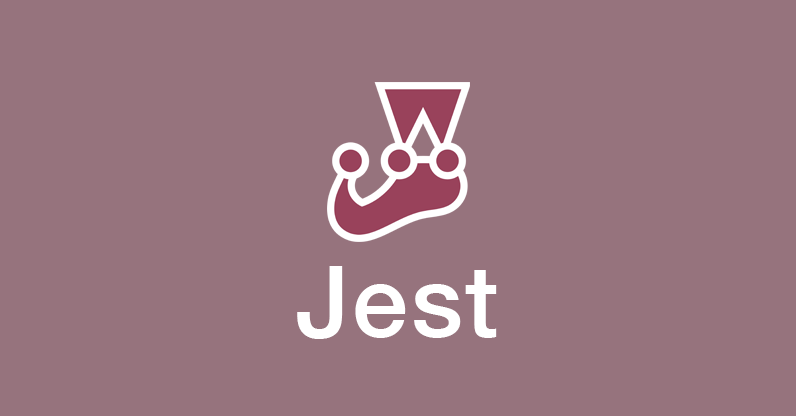
Source: jestjs.io
In this tutorial, we will write our first test using Jest.
What is Jest?
Jest is a delightful JavaScript Testing Framework with a focus on simplicity.
Jest is a fast, flexible, and feature-rich JavaScript testing library
that helps you write and run high-quality tests for your JavaScript applications like React, Vue, Angular, Express, and Node.
Why do I like Jest?
Because it works out of the box, you don't need anything to write tests just Jest
is enough. If you have ever worked with Mocha
then you know what I am talking about. If you use Mocha
then you need other libraries like chai
, sinon
.
Now we know what Jest is. Let's write our first test.
Prerequisite
Before following this tutorial, you have to make sure that you have basic knowledge of JavaScript
. Also, you will need to have a few tools installed on your computer
- Node
- npm or yarn
- Code editor (VSCode, Atom, etc)
Setup project
First, create a directory. If you prefer terminal
like me then use the following command otherwise use GUI.
1mkdir jest-tutorial
Now jest-tutorial
directory is created. Now go to that directory.
1cd jest-tutorial
Now we need to add packages to write tests. To add packages in our project we need to npm
.
1npm init -y
This will create a package.json
file. This file contains important metadata about the project, such as its name, version, description, author, dependencies, and more.
To write tests, we need to have the Jest
installed on our project. Let's install Jest
.
1npm install -D jest
We have installed Jest as a dev dependency. Now open our project in the code editor.
1code .
Now we can see our project in VSCode
.
Write the first test
We want to write tests, but what we are going to test? We need something to test. Let's write a sayHi
function so that we can test it. That function will take a name
and return a simple string like Hi name!
. That's it.
Create src
directory inside that create a file sayHi.js
.
1function sayHi(name) {2 return `Hi ${name}!`;3}45module.exports = sayHi;
Add the above code snippet to sayHi.js
. Now we have a function to tests. We will create a directory called tests
where we will put our all tests.
Create a tests
directory, inside that create a file sayHi.test.js
.
1const sayHi = require('../sayHi.js');23test('should say hi to John', () => {4 const result = sayHi('John');5 const expected = 'Hi john';67 expect(result).toBe(expected);8});
Add the above code snippet to sayHi.test.js
. Let's learn what's the above code doing.
Breakdown of test snippet
- test:
test
is a method which runs a test. This method accepts 3 argumentstest(name, fn, timeout)
.- name: It defines the name of the test. Actually, it is the description of what we are going to test.
- fn: It takes a function that contains the expectations to test.
- timeout: This one is optional.
timeout
specifying how long to wait before aborting a test. Default value is 5 seconds.
- expect:
expect
function is used every time when we want to test a value. - tobe:
tobe
is a matcher function. It is used to compare primitive values or to check the referential identity of object instances.
First, we are defining a test by giving the name should say hi to John
. Then we store the sayHi
function's return value in result
variable by calling the function. Then we store our expected value in expected
variable. In the last line, we are asserting whether our result
is equal to expected
or not.
Note - We can use it
instead of test
. They are same.
Are you curious about what the heck is .test.js
?
It is a file extension is commonly used for files containing automated tests for JavaScript code. We can also use .spec.js
but I prefer .test.js
.
Congrats! we have written our first test. Let's run our test.
Run test
To run the test, we have set npm spcript
for it with Jest
. Open package.json
file and inside that file, you will see test
script already present. We need to modify it.
1test: "jest"
Now open your terminal and run the script.
1npm run test
OOpps! our test failed.
But why? let's find out.
Update test
I have done this intentionally. There are two problems.
- The name is mismatched. (lowercase and uppercase)
!
is missing in our test.
Look at the screenshot carefully, you will find that problems are marked (Expected & Received)
. Let's update the test.
1const sayHi = require('../sayHi.js');23test('should sayHi to John', () => {4 const result = sayHi('John');5 const expected = 'Hi John!';67 expect(result).toBe(expected);8});
Run the test.
1npm run test
Hurrah! Our test is passed.
Did you notice something? In our current implementation, we have to run
npm run test
every time change something in out test file. Let's fix it.
Jest Watch
Again open package.json
file, inside script
object add another script for watching our tests.
1test:watch: "jest --watch"
Before running Jest
in watch mode, we need to initialize git
in our project otherwise it will not work.
1git -init
Now run the command.
1npm run test:watch
Now change something in test
file and save it and see the magic. It will immediately run the tests.
In watch mode, there are a couple of options available.
a
- will run all tests.f
- will run only failed tests. f to run only failed tests.p
- to filter by a filename regex pattern.t
- to filter by a test name regex pattern.q
- to quit watch mode.
I am highly encourage you to explore them.
Install Jest
extension to VSCode
There is another better way to get feedback about your tests. You will get notified about your test status while you are writing tests without running the test script. How it is possible? All you need is to install the Jest extension in your VSCode
. Install that extension, and use Jest With Pleasure :).
After installing that extension, beside every test you will get a tick
or cross
mark based on your test status (failed/success) like above image.
Summary
In this tutorial, we learned
- What is Jest
- How to write tests using Jest
- Jest watch mode
Now you know how to write tests. Congrats! Go and write tests for your current project. Best of luck.
Thanks for reading, I appreciate your time and effort. Another thing, If you feel it is valuable for others and you think your friends/colleagues need to know about this, Please share it with them. You can get the example code from my github repo.